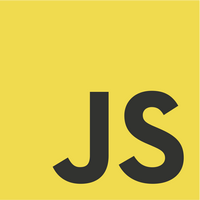
Javascript class kniznice
Javascript je prototype-base programovaci jazyk, jeho struktura tried je ina, ako v tradicnych C-like jazykoch. V clanku Uvod do Javascript OOP, sa docitate, ako stvorit jednoduchu triedu. Tato struktura mnohym nevyhovuje, pozrime sa na kniznice, ktore ponukaju prehladnejsiu syntax. Dalsie kniznice mozno doplnim casom.
Cisty Javascript, aby sme mali s cim porovnat kod:
function Person(name) {
this.name = name;
}
Person.prototype.getName = function() {
return this.name;
};
function Employee(name, salary) {
Person.call(this, name);
this.salary = salary;
}
Employee.prototype = Object.create(Person.prototype);
Employee.prototype.getSalary = function() {
return this.salary;
};
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
base2
https://code.google.com/p/base2/
var Person = Base.extend({
constructor: function(name) {
this.name = name;
},
name: "",
getName: function() {
return name;
}
});
var Employee = Person.extend({
constructor: function(name, salary) {
this.base(name);
this.salary = salary;
},
salary: "",
getSalary: function() {
return salary;
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
Classify
http://classify.petebrowne.com/
classify('Person', function() {
def('inicialize', function(name) {
this.name = name;
});
def('getName', function() {
return this.name;
});
});
classify(Person, 'Employee', function() {
def('inicialize', function(name, salary) {
this.callSuper(name);
this.salary = salary;
});
def('getSalary', function() {
return this.salary;
});
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
Classy
var Person = Class.$extend({
__init__: function(name) {
this.name = name;
},
getName: function() {
return this.name;
}
});
var Employee = Person.$extend({
__init__: function(name, salary) {
this.$super(name);
this.salary = salary;
},
getSalary: function() {
return this.salary;
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
dejavu
http://indigounited.com/dejavu/
var Person = dejavu.Class.declare({
_name: null,
initialize: function(name) {
this._name = name;
},
getName: function() {
return this._name;
}
});
var Employee = dejavu.Class.declare({
$extends: Person,
_salary: null,
initialize: function(name, salary) {
this.$super(name);
this._salary = salary;
},
getSalary: function() {
return this._salary;
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
Dojo Toolkit
http://dojotoolkit.org/reference-guide/dojo/_base/declare.html
var Person = dojo.declare(null, {
constructor: function(name) {
this.name = name;
},
getName: function() {
return this.name;
}
});
var Employee = dojo.declare(Person, {
constructor: function(name, salary) {
this.salary = salary;
},
getSalary: function() {
return this.salary;
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
Fiber
https://github.com/linkedin/Fiber/
var Person = Fiber.extend(function() {
return {
init: function(name) {
this.name = name;
},
getName: function() {
return this.name;
}
}
});
var Employee = Person.extend(function(base) {
return {
init: function(name, salary){
base.init.call(this, name);
},
getSalary: function(){
return this.salary;
}
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
John Resig
http://ejohn.org/blog/simple-javascript-inheritance/
var Person = Class.extend({
init: function(name) {
this.name = name;
},
getName: function() {
return this.name;
}
});
var Employee = Person.extend({
init: function(name, salary) {
this._super(name);
this.salary = salary;
},
getSalary: function() {
return this.salary;
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
Joose
Class("Person", {
has: {
name: {
is: "rw",
init: ""
}
},
methods: {
getName: function() {
return this.name;
}
}
});
Class("Employee", {
isa: Person,
has: {
salary: {
is: "rw"
}
},
methods: {
getSalary: function() {
return this.salary;
}
}
});
var person = new Person({ name: 'Jan' });
var employee = new Employee({ name: 'Jan', salary: 800 });
jsclass
var Person = new Class({
initialize: function(name) {
this.name = name;
},
getName: function() {
return this.name;
}
});
var Employee = new Class(Person, {
initialize: function(name, salary) {
this.callSuper(name);
this.salary = salary;
},
getSalary: function() {
return this.salary;
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
jsface
https://github.com/tnhu/jsface
var Person = Class({
constructor: function(name) {
this.name = name;
},
getName: function() {
return this.name;
}
});
var Employee = Class(Person, {
constructor: function(name, salary) {
this.$class.$super.call(this, name, age);
this.salary = salary;
},
getSalary: function() {
return this.salary;
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
Klass
var Person = klass(function (name) {
this.name = name
})
.methods({
getName: function () {
return this.name;
}
});
var Employee = Person.extend(function (name, salary) {
this.salary = salary;
})
.methods({
getSalary: function() {
return this.salary;
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
MooTools
http://mootools.net/docs/core/Class/Class/
var Person = new Class({
initialize: function(name) {
this.name = name;
},
getName: function() {
return this.name;
}
});
var Employee = new Class({
Extends: Person,
initialize: function(name, salary) {
this.parent(name);
this.salary = salary;
},
getSalary: function() {
return this.salary;
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
Prototype
http://prototypejs.org/learn/class-inheritance/
var Person = Class.create({
initialize: function(name) {
this.name = name;
},
getName: function() {
return this.name;
}
});
var Employee = Class.create(Person, {
initialize: function($super, name, salary) {
$super(name);
this.salary = salary;
},
getSalary: function() {
return this.salary;
}
});
var person = new Person('Jan');
var employee = new Employee('Jan', 800);
Vyvolanie metod
person.getName(); // Jan
employee.getName(); // Jan
employee.getSalary(); // 800
Overenie dedicnosti
console.log(employee instanceof Person); // true
console.log(employee instanceof Employee); // true